A Detailed Guide on State Management in React Native
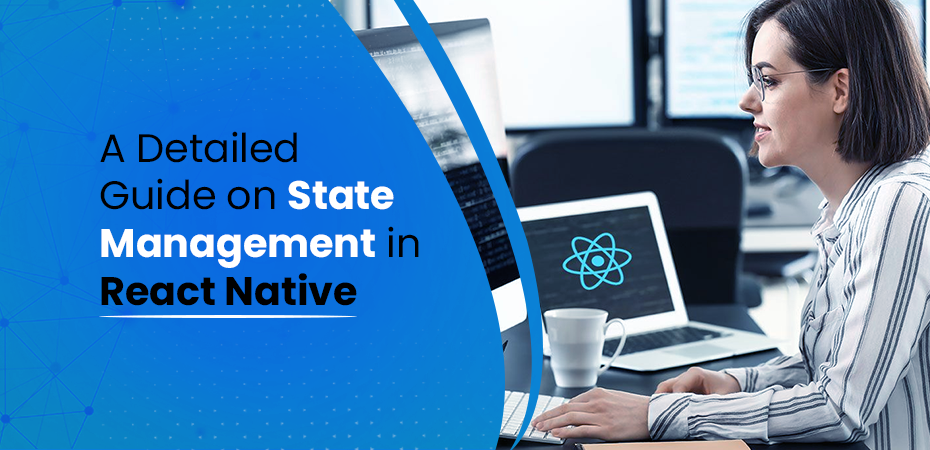
State management is a cornerstone of building dynamic, responsive, and efficient applications in React Native. As your application grows in complexity, understanding and implementing effective React Native state management becomes crucial. This guide will explore the fundamentals of state management in React Native, the various techniques available, and how to choose the best approach for your application.
What is State in React?
In React, state refers to the data that determines the behavior and rendering of components. When this data changes, React automatically updates the affected components, providing a dynamic and interactive user experience. Think of state as the memory of your component, holding information that can change over time, such as user inputs, fetched data, or UI elements that respond to user interactions.
React State: The Basics
Using state in React begins with the React use State hook or, in class components, with this.state. For instance:
const [count, setCount] = useState(0); // useState in a functional component
// Or in a class component:
this.state = {
count: 0,
};
When you need to change the state, you use React setState in class components or the setCount function in functional components:
// Functional component
setCount(count + 1);
// Class component
this.setState({ count: this.state.count + 1 });
This change triggers a re-render, ensuring the UI reflects the updated state.
Why State Management is Crucial?
Managing state is crucial because it directly affects the behavior and rendering of your components. As your application grows, managing state can become increasingly complex. Poor state management can lead to bugs, unpredictable behavior, and performance issues. Therefore, understanding and implementing effective state management strategies is essential for building reliable and maintainable React Native applications.
SetState React: Managing State Changes Efficiently
When working with React, understanding how to manage state changes is crucial. The Set State React method plays a central role in this process. It allows you to update the state of a component, triggering a re-render to reflect the changes in the UI.
For instance, when a user interacts with an input field or a button, React JS setState can be used to update the data and ensure the interface remains consistent. Mastering React State setState is essential for handling dynamic content and creating responsive applications.
ReactJS Props vs. State in React
Props are another way to pass data in React, but unlike state, they are read-only. Props are used to send data from a parent component to a child component, allowing the child to render content based on the parent’s data. For instance, you might send state as props in React to a child component:
<ChildComponent count={this.state.count} />
In this example, the count state is passed down as a prop to ChildComponent, which can now use it in rendering.
Challenges in State Management
As applications grow, managing state can become complex, especially when different parts of the application need access to the same state. Here are some common challenges:
Component Resets State of Parent
Scenario: A common issue arises when a child component resets the state of its parent, causing unexpected behavior. Properly handling state updates is crucial to avoid such issues.
Access Parent State in Child React
Scenario: Sometimes, you need to access or modify the parent’s state within a child component. This can be tricky and often requires carefully structuring your state management.
State Management Across Components
Scenario: As your app grows, managing state across multiple components can become challenging. Without a structured approach, your codebase can quickly become hard to maintain.
React State Management Techniques
React’s Built-in setState and useState
Use Case: For simple, localized state management, React’s built-in tools like useState and this.setState are sufficient. They allow you to manage state within individual components easily.
Example
const [inputValue, setInputValue] = useState(”);
const handleInputChange = (event) => {
setInputValue(event.target.value);
};
Here, inputValue holds the current value of the input, and setInputValue updates the state as the user types.
Lifting State Up
Use Case: When multiple components need to share the same state, lift the state to their common ancestor and pass it down as props.
Example
const ParentComponent = () => {
const [sharedState, setSharedState] = useState(”);
return (
<div>
<ChildComponent1 sharedState={sharedState} setSharedState={setSharedState} />
<ChildComponent2 sharedState={sharedState} />
</div>
);
};
React Context API
Use Case: For applications with more complex state needs that span multiple components, the Context API allows you to create global state that can be accessed and updated from anywhere in your component tree.
Example
const MyContext = React.createContext();
const MyProvider = ({ children }) => {
const [state, setState] = useState(‘default value’);
return (
<MyContext.Provider value={{ state, setState }}>
{children}
</MyContext.Provider>
);
};
const MyComponent = () => {
const { state, setState } = useContext(MyContext);
return <div>{state}</div>;
};
Redux: A Comprehensive State Management Library
Use Case: For large applications with complex state interactions, Redux provides a predictable state container that helps manage application state consistently.
Key Features
- Single Source of Truth: Redux stores the entire application state in a single object, ensuring that every part of your app can access the most up-to-date state.
- Predictable State Updates: Actions and reducers in Redux provide a clear and predictable way to update the state.
- DevTools: Redux DevTools enable time-travel debugging, making it easier to trace state changes and diagnose issues.
Other React State Management Libraries
- MobX: A library that makes state management simple and scalable using observables.
- Recoil: Developed by Facebook, Recoil offers a flexible way to manage state with minimal boilerplate.
- Zustand: A small, fast, and scalable state management solution that works well with React hooks.
Best Practices for State Management in React
1. Keep State Local When Possible
Recommendation: Avoid lifting state higher in the component tree unless necessary. Local state is simpler and more efficient to manage.
2. Choose the Right State Management Tool
Recommendation: Use built-in React tools for simple applications, and opt for libraries like Redux or MobX for more complex needs.
3. Avoid Over-Optimization
Recommendation: Start with React’s basic state management techniques and only move to more complex solutions if your application requires it.
4. Understand React’s Rendering Behavior
Recommendation: Learn how React decides when to re-render components. Tools like React.memo can help prevent unnecessary renders.
5. Document Your State Management Approach
Recommendation: Keep clear documentation of your state management strategy, especially when working in a team. This helps maintain consistency and makes the codebase easier to understand.
Case Study: Implementing State Management in a Sample App
Overview of the Sample App
Let’s consider a simple shopping cart app where users can add, remove, and update items.
Step-by-Step Implementation
- Setting up the App: Create a basic React Native project and set up the necessary state management tool, such as Redux.
- Implementing State Management: Use Redux to manage the global state of the cart, including actions like addItem, removeItem, and updateItem.
- Key Considerations: Pay attention to performance and scalability, especially as the app grows.// Example action in Reduxconst addItem = item => ({
type: ‘ADD_ITEM’,
payload: item,
});
// Example reducer
function cartReducer(state = [], action) {
switch (action.type) {
case ‘ADD_ITEM’:
return […state, action.payload];
case ‘REMOVE_ITEM’:
return state.filter(item => item.id !== action.payload.id);
case ‘UPDATE_ITEM’:
return state.map(item =>
item.id === action.payload.id ? action.payload : item
);
default:
return state;
}
}
Conclusion
Effective state management is key to building robust, scalable React Native applications. Whether you’re managing state locally with useState or using advanced libraries like Redux, understanding the fundamentals of state management will help you create more maintainable and efficient applications. As you continue to develop your React Native projects, keep these principles in mind to ensure your state management approach aligns with your app’s needs and growth.
Experiment with different state management solutions in your projects and see what works best for you. Don’t hesitate to share your experiences and challenges with the community!