A Detailed Guide on State Management in React Native
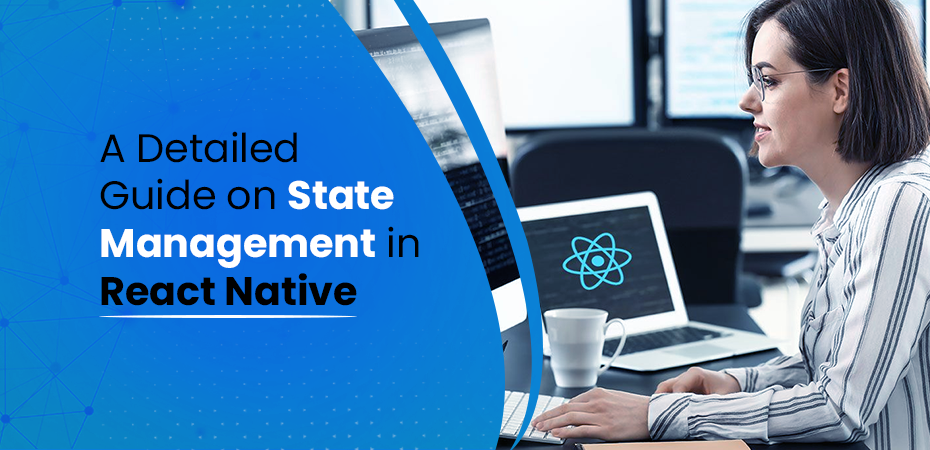
When building complex React Native applications, managing state and handling multiple users and screens can become challenging with just the built-in tools. However, state management in React Native allows developers to create robust and efficient apps by enabling smooth data flow and sharing across components.
Key Takeaways from This Article:
- Understand the significance of effective state management in React Native.
- Explore various built-in tools and popular libraries for managing state.
- Learn best practices for optimizing performance.
- Gain practical insights through implementation examples using different libraries.
Understanding State Management in React Native
Definition and Significance of Effective State Management for Complex Applications
When building complex React Native applications, understanding state management is crucial. State management refers to the practice of handling the state—a collection of variables that determine the behavior and appearance of the app at any given time. Effective state management ensures smooth user experiences by consistently reflecting UI changes and managing data flow efficiently.
In large applications, improper state management can lead to:
- Inconsistent UI states: Components may not reflect the current data accurately.
- Data redundancy: Multiple components may store the same data, leading to synchronization issues.
- Complex debugging: Tracking down bugs becomes harder as the state logic spreads across multiple components.
A robust state management strategy mitigates these issues by providing a structured approach to handle state updates and sharing across components.
Challenges in State Management
As applications grow, managing state can become complex, especially when different parts of the application need access to the same state. Here are some common challenges:
Component Resets State of Parent
Scenario: A common issue arises when a child component resets the state of its parent, causing unexpected behavior. Properly handling state updates is crucial to avoid such issues.
Access Parent State in Child React
Scenario: Sometimes, you need to access or modify the parent’s state within a child component. This can be tricky and often requires carefully structuring your state management.
State Management Across Components
Scenario: As your app grows, managing state across multiple components can become challenging. Without a structured approach, your codebase can quickly become hard to maintain.
The Difference Between Global and Local State Management
Understanding React Native state management involves distinguishing between global and local states.
Local State Management
Local state management refers to managing state within a single component. It is suitable for simple, isolated component behaviors where the state does not need to be shared with other components.
Local states are implemented using tools like React’s use State, a built-in hook.
Global State Management
Global state management involves sharing state across multiple components. This approach is essential for handling data that needs to be accessible throughout the application, such as user authentication status or application settings.
Global states can be implemented using tools like Context API or third-party libraries like Redux.
Choosing between global and local state depends on the scope and complexity of your application. Local states are ideal for encapsulated component-specific logic, while global states are necessary when multiple components need access to shared data.
How Built-in Tools Like React Native’s useState Work
React provides built-in hooks such as useState to manage local component states effectively.
javascript import React, { useState } from ‘react’; import { View, Button, Text } from ‘react-native’;
const Counter = () => { const [count, setCount] = useState(0);
return (
<View>
<Text>{count}</Text>
<Button title=”Increment” onPress={() => setCount(count + 1)} />
</View>
);
};
In this example:
- use State in React initializes the count variable with a value of 0.
- setCount updates count, triggering a re-render of the component with the new value.
Effective React state management ensures seamless data flow and consistent UI updates, making it indispensable for developing complex applications. Balancing between local and global state strategies helps maintain clarity and efficiency in your codebase.
Before hooks like useState were introduced, React Native used a method called React setState to manage state in class components. setState in React allows you to update the state of your component, which will then trigger a re-render of the UI. Mastering React State setState is essential for handling dynamic content and creating responsive applications.
Built-in State Management Tools in React Native
Managing state and handling multiple users and screens can become challenging with just the built-in tools. However, state management in React Native allows developers to create robust and efficient apps by enabling smooth data flow and sharing across components. As a business owner or entrepreneur, understanding these tools will empower you to make informed decisions regarding your app’s architecture.
Context API
The Context API is a powerful feature that allows for sharing of state across different components without the need to send state as props react manually at every level. It is particularly useful for global state management, such as user authentication or theme settings.
Role
It provides a way to pass data through the component tree without having to pass props down manually at every level.
Usage
Create a Context using React.createContext(), then use a Provider component at the top level of your app to provide the state, and Consumers or useContext hook in child components to access that state.
SetState
setState is a method used in React Native class components to update the component’s state. When the state changes, setState triggers the UI to re-render with the updated values.
Usage
set State in React allows you to update the state within a class component, which automatically triggers a UI re-render.
Key Features of React set State:
- Updates State: this.setState() allows you to change the state and re-render the UI with the updated state.
- State Access: this.state is used to access the current state of the component.
Pros of Using setState:
- Simple for Class Components: If you’re working with class components, setState provides an easy way to manage state.
- Partial Updates: You only need to update the part of the state that changes, leaving the rest intact.
Cons of Using setState:
- Limited to Class Components: setState is only usable within class components, whereas hooks like useState work with functional components, which are now more popular.
- More Verbose: Managing state in class components is more verbose compared to using hooks like useState in functional components.
useState
The useState hook allows you to add state to functional components. It is ideal for managing local component states such as form inputs or toggles.
Syntax
javascript const [state, setState] = useState(initialState);
Pros
- Simple syntax, easy to understand and implement.
- Great for handling simple local states.
Cons
- Not suitable for complex state logic or global state management.
useReducer
The useReducer hook is another way to manage local state within a component. It is more suited for complex state logic where multiple sub-values are involved.
Syntax
javascript const [state, dispatch] = useReducer(reducer, initialState);
Pros
- Ideal for complex state manipulations.
- Helps keep the logic contained within the reducer function.
Cons
- More boilerplate code compared to useState.
- Can be overkill for simple state management needs.
Pros and Cons of Using Built-in Tools
Pros:
- Integrated into React Native, no additional libraries needed.
- Simple setup and usage.
- Good for small-scale applications with straightforward state requirements.
Cons:
- Limited scalability for large applications.
- Can lead to prop-drilling issues when not used with Context API.
- Managing state across multiple screens and user interactions can become cumbersome.
Understanding these built-in tools sets the foundation for effective state management in your React Native applications. The choice between them depends on the complexity of your application and specific requirements. To further enhance your development workflow, consider exploring some of the best React Native debugging tools available in 2024. These tools can significantly improve your bug detection and resolution process, making your development experience smoother and more efficient.
Popular React State Management Libraries
When building complex applications with React Native, managing state and handling multiple users and screens can become challenging with just the built-in tools. However, understanding state management in React Native allows developers to create robust and efficient apps by enabling smooth data flow and sharing across components.
As a business owner or entrepreneur, it’s important to understand how React Native state management simplifies the development of enterprise-level applications. In this section, we’ll explore popular libraries that enhance state management in React Native.
MobX: A Reactive Approach to State Management in React Native Apps
Introduction to MobX
MobX is a reactive state management library that leverages observables to manage application states. Unlike some other libraries that require boilerplate code, MobX focuses on simplicity and reactivity, allowing developers to concentrate on what matters most—building their applications.
Key Features of MobX:
- Observables: Track the state and automatically update components when the state changes.
- Actions: Encapsulate state modifications, ensuring that all changes are trackable and predictable.
- Reactions: Automatically perform side effects based on state changes.
- Computed Values: Derive values from existing state without redundant calculations.
Reactive Programming with MobX
MobX employs reactive programming principles, making it different from traditional imperative programming. This approach ensures that any change in the observable state triggers automatic updates in the UI, creating a seamless user experience.
javascript import { makeAutoObservable } from “mobx”;
class TodoStore { todos = [];
constructor() { makeAutoObservable(this); }
addTodo = (todo) => { this.todos.push(todo); };
get completedTodosCount() { return this.todos.filter((todo) => todo.completed).length; } }
const todoStore = new TodoStore();
In this example:
- todos array is an observable.
- addTodo action modifies the todos array.
- completedTodosCount is a computed value derived from todos.
Comparison with Redux
Redux is often considered the go-to solution for complex React Native applications due to its predictable state container model. It uses actions and reducers to manage state changes in a predictable manner. However, Redux can be verbose and requires significant boilerplate code.
Pros of Redux:
- Predictability: State transitions are explicit and predictable.
- Middleware Support: Extensive middleware ecosystem for handling asynchronous actions.
Cons of Redux:
- Boilerplate Code: Requires defining actions, reducers, and store setup.
- Learning Curve: Steeper learning curve compared to simpler libraries like MobX.
Pros of MobX:
- Simplicity: Less boilerplate code; developers can focus on application logic.
- Reactivity: Automatic updates ensure UI consistency without manual intervention.
Cons of MobX:
- Implicit Updates: The automatic nature might make debugging more challenging if not properly understood.
- Less Predictable Flow: Compared to Redux’s explicit flow of actions and reducers.
Choosing Between MobX and Redux
Choosing between MobX and Redux depends on your project needs:
- If you prioritize simplicity and want to avoid excessive boilerplate code, MobX might be the better choice.
- If you require strict predictability in your state transitions and are comfortable with the additional complexity of actions and reducers, Redux could be more suitable.
Zustand: A Lightweight State Management Solution for Developers Seeking Simplicity
Zustand is an open-source state management library designed to deliver simplicity and efficiency. Unlike more complex libraries such as Redux, Zustand offers a minimalistic API that prioritizes ease of use and developer experience.
Overview of Zustand’s Features
- Minimalistic API: Zustand’s API is straightforward, allowing developers to set up and manage state with minimal boilerplate code.
- Performance Optimized: The library is crafted to be highly performant, ensuring rapid updates and efficient state management without unnecessary re-renders.
- Flexible State Configuration: Zustand provides flexibility in defining state slices, making it easier to manage different parts of your application’s state independently.
Benefits for Developers Looking for a Minimalistic Approach
- Ease of Integration: Zustand can be easily integrated into existing React Native projects without extensive refactoring or setup.
- Reduced Boilerplate: Unlike Redux, which requires the creation of actions and reducers, Zustand simplifies the process by using a hook-based API.
- Scalability: While designed for simplicity, Zustand scales well with application complexity, making it suitable for both small and large-scale projects.
javascript // Example of setting up a store in Zustand import create from ‘zustand’;
const useStore = create(set => ({ bears: 0, increasePopulation: () => set(state => ({ bears: state.bears + 1 })), }));
// Usage in a component function BearCounter() { const bears = useStore(state => state.bears); const increasePopulation = useStore(state => state.increasePopulation);
return ( {bears} Bears Increase population ); }
For developers accustomed to the structure imposed by Redux’s actions and reducers, transitioning to Zustand can significantly streamline workflows. By eliminating the need for boilerplate code, Zustand allows developers to focus on building features rather than managing intricate state configurations. This minimalistic approach aligns well with projects that demand quick iterations and efficient development cycles.
In comparison to tools like Redux Toolkit—which aims to simplify Redux by providing abstractions over its core functionalities—Zustand naturally caters to those seeking an even lighter solution without compromising performance or scalability.
Recoil: Atom-Based State Management for Efficient Data Flow Between Components
Recoil is an innovative state management library that provides a solution for complex state management in React Native applications. Unlike traditional libraries like Redux, Recoil introduces atoms and selectors to handle shared state more efficiently.
Key Concepts of Recoil
- Atoms: Atoms are units of state. They can be read from or written to, and any component that subscribes to an atom will re-render when the atom’s state changes.
javascript import { atom } from ‘recoil’;
const textState = atom({ key: ‘textState’, // unique ID default: ”, // default value (aka initial value) });
- Selectors: Selectors allow you to compute derived state based on atoms. They are pure functions that transform the state and can be used to derive values or perform calculations.
javascript import { selector } from ‘recoil’;
const charCountState = selector({ key: ‘charCountState’, get: ({get}) => { const text = get(textState); return text.length; }, });
Benefits of Using Recoil
- Efficient Data Flow: By utilizing atoms and selectors, Recoil ensures efficient data flow between components. This approach minimizes unnecessary re-renders and optimizes performance.
- Flexibility: Recoil’s API is designed to be minimalistic yet flexible, allowing developers to manage global and local states seamlessly.
- Ease of Integration: Integrating Recoil into existing React Native projects is straightforward, which makes it a popular choice among developers seeking simplicity without sacrificing functionality.
Recoil presents a modern approach to state management by addressing some of the common challenges faced with traditional libraries like Redux. Its architecture promotes efficient data handling and simplifies the complexity associated with managing large-scale React Native applications.
Valtio: Simplifying Mutable Data Structures While Maintaining Reactivity in Your Applications
Valtio offers a unique approach to state management in React Native by simplifying mutable data structures while maintaining reactivity.
Key Features of Valtio:
- Mutable State: Unlike libraries that enforce immutability, Valtio allows you to work with mutable state directly. This can be more intuitive for developers who are familiar with working in an imperative style.
- Automatic Reactivity: Changes to the state automatically trigger re-renders in your components, maintaining a seamless user experience without requiring manual updates.
- Minimal Boilerplate: Valtio reduces the boilerplate code needed for managing states, making it easier and faster to implement.
Benefits of Using Valtio:
- Simplicity: Valtio’s API is straightforward, allowing developers to focus more on building features rather than managing complex state logic.
- Flexibility: Mutable states can be easier to manage and understand, especially for those who are new to reactive programming.
- Performance: By leveraging proxies, Valtio ensures efficient reactivity without the overhead associated with other state management solutions.
To illustrate how Valtio works, consider this simple example:
javascript import { proxy, useSnapshot } from ‘valtio’;
const state = proxy({ count: 0 });
function Counter() { const snapshot = useSnapshot(state); return ( {snapshot.count} <button onClick={() => ++state.count}>Increment ); }
In this example:
- proxy creates a mutable state object.
- useSnapshot subscribes the component to changes in the state.
- Incrementing the count directly modifies the state and triggers a re-render.
Valtio stands out among popular libraries due to its simplicity and performance benefits. Unlike Redux, which uses actions and reducers within a predictable state container, Valtio focuses on minimalism and ease of use. For developers overwhelmed by the complexity of Redux or searching for a lightweight alternative like Zustand or MobX’s observables, Valtio provides an attractive solution.
Choosing the Right State Management Tool for Your Project Needs
Selecting the appropriate state management tool can significantly impact the efficiency and maintainability of your React Native application. Here are some factors to consider when choosing the right tool:
Application Complexity
- Simple Applications: For small projects with minimal state management requirements, built-in tools like useState and useReducer might suffice. These hooks offer straightforward solutions without introducing additional complexity.
- Moderate Complexity: For applications that require more advanced state sharing across multiple components, Context API combined with hooks can be effective. However, managing larger states might still be challenging.
- Highly Complex Applications: When dealing with intricate state logic or extensive data flow, dedicated libraries such as Redux, MobX, Recoil, Zustand, or Valtio become essential. These tools offer robust frameworks for managing complex states efficiently.
Team Expertise
- Familiarity with Tools: The team’s existing knowledge and experience play a crucial role in tool selection. Teams well-versed in Redux might prefer sticking with it despite its verbosity due to their familiarity with its ecosystem.
- Learning Curve: Tools like MobX and Zustand are known for their simplicity and shorter learning curves compared to Redux. Recoil’s atom-based approach might also be easier for teams new to state management in React Native.
- Community Support: Opting for tools with strong community support ensures access to a wealth of resources, documentation, and third-party libraries. Redux leads in this aspect with extensive community contributions.
Future Scalability Needs
- Growth Considerations: Planning for future growth is essential when selecting a state management strategy. Redux offers excellent scalability due to its predictable state container and middleware support.
- Performance Optimization: Tools like Recoil and Valtio provide efficient ways to manage reactivity and optimize performance through selective updates and memoization techniques.
- Maintainability: Assessing how easy it is to maintain codebases as they grow is critical. MobX’s reactive approach can simplify updates but may introduce challenges if not structured properly.
Choosing the right state management tool for your project involves balancing these factors based on your specific needs and team capabilities.
Best Practices for Optimizing Performance
Implementing effective state management strategies is crucial for optimizing performance in complex React Native applications.
This section will delve into recommended practices, tips on optimizing performance, and common pitfalls to avoid when managing states.
Recommended Practices
- Minimize State Duplication: Avoid duplicating state across multiple components. This reduces complexity and prevents inconsistencies.
- Use Local State for UI-Related Data: Reserve global state for data that needs sharing across multiple components. Use local state (useState) for component-specific data.
- Leverage Memoization: Use React.memo and useMemo to prevent unnecessary re-renders of components.
- Immutable Data Structures: Adopt immutable data structures to ensure predictable state updates and easier debugging.
- Batch State Updates: Take advantage of batching in React to minimize the number of re-renders during multiple state updates.
Tips on Optimizing Performance
- Effective use of Context API: Properly structure the Context API to avoid unnecessary re-renders. Consider splitting contexts if different parts of the tree require different data.
- Throttling and Debouncing: Implement throttling or debouncing techniques for event handlers like scrolling or resizing to enhance performance.
- Code Splitting: Utilize dynamic imports and lazy loading to split code bundles, reducing the initial load time.
Common Pitfalls to Avoid
- Overusing Global State: Not everything needs to live in global state. Overusing can lead to excessive re-renders and performance issues.
- Neglecting Cleanup Effects: Ensure proper cleanup of side effects using useEffect cleanup functions to prevent memory leaks.
- Ignoring Component Hierarchy Complexity: Deeply nested components can be hard to manage and debug. Aim for a flatter component hierarchy when possible.
By adhering to these best practices for optimizing performance through effective state management strategies, you can enhance user experiences and boost application performance using the right tools.
// store.js import create from ‘zustand’;
const useStore = create(set => ({ count: 0, increment: () => set(state => ({ count: state.count + 1 })), decrement: () => set(state => ({ count: state.count – 1 })) }));
export default useStore;
Connect Store to Component: javascript // App.js import React from ‘react’; import useStore from ‘./store’;
const Counter = () => { const { count, increment, decrement } = useStore();
return (
<div>
<h1>{count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
const App = () => ( );
export default App;
Implementing State Management with Recoil
Recoil provides a way to manage state that is both global and local to components.
- Install Recoil: shell npm install recoil
- Create Atom: javascript // atoms.js import { atom } from ‘recoil’; export const countState = atom({ key: ‘countState’, default: 0, });
- Connect Atom to Component: javascript // App.js import React from ‘react’; import { RecoilRoot, useRecoilState } from ‘recoil’; import { countState } from ‘./atoms’;
const Counter = () => { const [count, setCount] = useRecoilState(countState);
return (
<div>
<h1>{count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount(count – 1)}>Decrement</button>
</div>
);
};
const App = () => ( );
export default App;
These examples demonstrate how different libraries can be used for state management in React Native applications. Each library has its own strengths and use cases, so the choice depends on the specific requirements of your project.
Conclusion
The importance of effective state management in React Native development cannot be overstated. As applications grow in complexity, the need for robust state management solutions becomes more critical. This is where understanding how React Native state management simplifies the development of enterprise-level applications comes into play, preparing developers to build scalable, efficient, and high-performing apps.
As we look towards the future, it’s also important to consider the broader landscape of app development. For instance, comparing frameworks like React Native vs Swift or Xamarin vs React Native can provide valuable insights into which technology might be best suited for specific project needs.