Best Tips to Improve React Native Performance
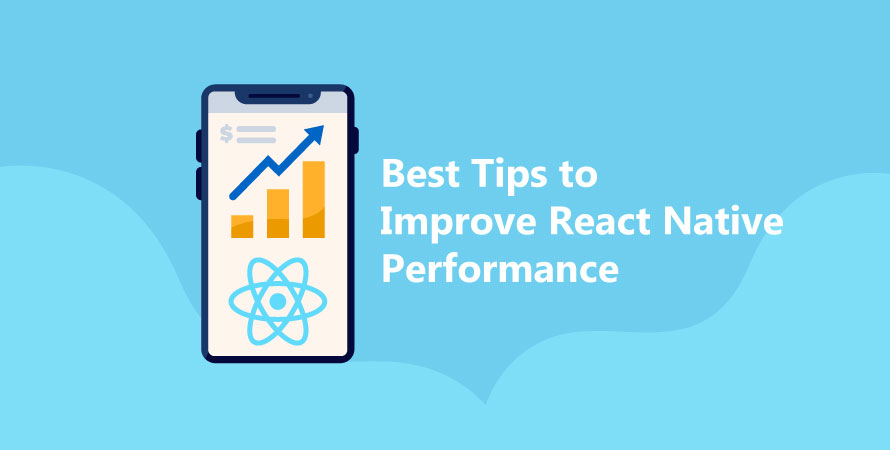
React Native is a popular framework that enables developers to build mobile applications using JavaScript and React. Its significance in app development lies in its ability to create high-quality apps for both iOS and Android platforms from a single codebase, significantly reducing development time and costs. The React Native launch date was 26 March 2015.
Android app performance optimization plays a crucial role in ensuring a smooth user experience and the overall success of an application. Poor performance can lead to slow load times, unresponsive interfaces, and crashes, which can frustrate users and result in low retention rates.
Optimizing React Native apps for better React performance encompasses several key areas:
- Rendering Speed: Ensuring that your app’s UI updates quickly to provide a seamless user experience.
- Memory Usage: Efficiently managing memory to prevent leaks and slowdowns.
- Network Requests: Minimizing the impact of network operations on your app’s responsiveness.
This article will delve into practical tips for improving React Native performance and launching your app faster. Each section will cover specific techniques, such as:
- Memoization
- Virtualized lists
- Image caching
- Debouncing actions
- Bundle size optimization
- Leveraging native modules
- Optimizing network requests
- Keeping up with the latest updates
Use Memoization to Minimize Re-renders
Memoization is a powerful optimization technique used in React Native to minimize unnecessary re-renders of components, which can significantly improve app performance and responsiveness.
What is Memoization?
Memoization involves caching the results of expensive function calls and reusing those cached results when the same inputs occur again. In the context of React Native, memoization can prevent components from re-rendering unless their props or state have changed, reducing the computational overhead.
Benefits of Memoization
Implementing memoization techniques in your React Native app offers several benefits:
- Reduced Re-renders: By preventing unnecessary re-renders, memoization helps maintain smooth and glitch-free user interactions.
- Improved Responsiveness: With fewer components needing to re-render, the app becomes more responsive and efficient.
- Optimized Performance: Less computational work translates to better overall app performance, contributing to faster load times and a more seamless user experience.
Optimize Memory Usage with Virtualized Lists
Efficient rendering of large datasets is crucial for maintaining optimal application performance. This is where the concept of virtualized lists comes into play in React Native best practices. The VirtualizedList component is designed specifically to handle long lists by only rendering items currently visible on the screen, thereby minimizing memory consumption.
Benefits of Virtualized Lists
- Reduced Memory Usage: By only rendering items that are in view, virtualized lists significantly cut down on memory usage. This approach avoids loading all list items into memory at once, which can be particularly beneficial when dealing with extensive datasets.
- Enhanced Scrolling Performance: Using virtualized lists improves scrolling performance because the app doesn’t have to process every single item in a large list. By this, you will learn how would you optimize your app. This results in smoother and faster response times.
Implementing Virtualized Lists
React Native game offers several components that implement virtualization:
- FlatList: Ideal for simple, flat list data.
- SectionList: Useful for lists grouped into sections.
- VirtualizedList: Provides a more customizable approach if FlatList or SectionList do not meet specific needs.
Here’s a basic example of using FlatList:
jsx import React from ‘react’; import { FlatList, Text, View } from ‘react-native’;
const data = Array.from({ length: 1000 }).map ((_, index) => ({ key: ${index}, value: Item ${index} }));
const renderItem = ({ item }) => ( {item.value} );
const MyVirtualizedList = () => ( <FlatList data={data} renderItem={renderItem} keyExtractor={item => item.key} /> );
export default MyVirtualizedList;
In this example:
- The data array contains 1,000 items.
- The renderItem function specifies how each item should be rendered.
- The keyExtractor property is used to ensure each item has a unique key.
Adopting these components can lead to substantial React performance optimization enhancements by efficiently managing memory and ensuring smooth list scrolling.
Improve Image Loading Speed with Caching Libraries
Loading images efficiently in React Native apps can be challenging. Large images or a considerable number of images can slow down the app, leading to a poor user experience. Caching plays a critical role in addressing these challenges by storing frequently accessed images locally, reducing the need to re-fetch them from remote servers.
Challenges of Image Loading in React Native
- Network Latency: Slow network connections can delay image loading.
- Memory Usage: Large images consume significant memory, potentially leading to performance bottlenecks.
- Re-fetching: Without caching, the same image might be fetched multiple times, wasting bandwidth and increasing load times.
Recommended Library: react-native-fast-image
To tackle these issues, using a dedicated library like react-native-fast-image is highly recommended. This library offers robust image caching mechanisms and optimized image loading capabilities.
Key Features of react-native-fast-image
- Aggressive Caching: The system caches images in both memory and on disk.
- Priority-based Loading: Load important images first to minimize React Native screen time.
- Preloading: Preload images before they are displayed on the React-native screen.
Implement Debouncing for Performance-sensitive Actions
Debouncing is a technique used to limit the rate at which a function executes, ensuring it only runs after a specified delay period has passed since the last invocation. This approach is particularly useful in React optimization to handle frequent user actions more efficiently, such as button clicks or text input events.
Benefits of Debouncing
Incorporating debouncing helps to:
- Prevent Excessive Calls: By delaying function execution, debouncing reduces the number of times a function is called in quick succession.
- Improve App Responsiveness: Minimizing unnecessary function calls enhances the overall responsiveness and performance of React Native app.
Implementation Examples
Using Custom Hooks
Create a custom hook to debounce user actions:
javascript import { useState, useEffect } from ‘react’;
const useDebounce = (value, delay) => { const [debouncedValue, setDebouncedValue] = useState(value);
useEffect(() => { const handler = setTimeout(() => { setDebouncedValue(value); }, delay);
return () => {
clearTimeout(handler);
};
}, [value, delay]);
return debouncedValue; };
export default useDebounce;
Usage in a component:
javascript import React, { useState } from ‘react’; import useDebounce from ‘./useDebounce’;
const SearchComponent = () => { const [query, setQuery] = useState(”); const debouncedQuery = useDebounce(query, 500);
useEffect(() => { if (debouncedQuery) { // Perform search with debounced query } }, [debouncedQuery]);
return ( <input type=”text” value={query} onChange={(e) => setQuery(e.target.value)} placeholder=”Search…” /> ); };
Utilizing Third-party Libraries
Libraries like lodash offer built-in support for debouncing functions:
javascript import React from ‘react’; import _ from ‘lodash’;
const SearchComponent = () => { const handleSearch = _.debounce((query) => { // Perform search with debounced query }, 500);
return ( <input type=”text” onChange={(e) => handleSearch(e.target.value)} placeholder=”Search…” /> ); };
Both custom hooks and third-party libraries provide effective ways to implement debouncing in React Native projects, enhancing performance by managing frequent user actions more efficiently.
Optimize Bundle Size for Faster App Loading
Reducing bundle size is crucial for improving the initial loading speed of React Native apps. A smaller bundle means less code to download, parse, and execute when the app starts, leading to quicker launch times and a smoother user experience.
Techniques for Bundle Size Optimization
Code Splitting: This technique involves breaking down your web application into smaller chunks that can be loaded on demand. Instead of loading the entire application at once, you load only the necessary parts initially, speeding up the initial load time.
Dynamic Imports: By using dynamic imports, you can defer the loading of certain modules until they are needed. This method reduces the initial bundle size and ensures that only essential code is loaded at startup.
javascript import(/* webpackChunkName: “myComponent” */ ‘./MyComponent’) .then(module => { const MyComponent = module.default; // Use MyComponent }) .catch(err => { console.error(‘Failed to load MyComponent’, err); });
Boost Performance with Native Modules and Components
Integrating Native Modules and Native Components into your React Native application can significantly boost performance by bridging the gap between JavaScript and native code. Native Modules are used to call platform-specific functionalities that may not be available in the React Native library, while Native Components allow you to create custom UI components with native behavior.
Benefits of Using Native Modules
By utilizing Native Modules, you can offload CPU-intensive tasks directly to the underlying platform. You can learn how to put apps on high performance through your mobile device. This capability is particularly useful for operations like:
- Image Processing: Offload complex image manipulations to native libraries which are usually more efficient.
- Background Tasks: Handle background processing more efficiently by leveraging native services.
- Hardware Access: Directly interact with device hardware such as cameras and sensors.
Best Practices for Working with Third-party SDKs
When integrating third-party SDKs using Native Modules and Components, consider the following best practices:
- Use Well-maintained Packages: Ensure that the SDK is regularly updated and well-documented.
- Code Reviews: Conduct thorough code reviews for security vulnerabilities and performance bottlenecks.
- Consistent APIs: Maintain consistency between native module APIs and React Native APIs to ensure seamless integration.
Example: Integrating a Third-party SDK
Here’s a simple example of how you might integrate a third-party SDK using a Native Module:
javascript // AndroidModule.java package com.yourapp;
import com.facebook.react.bridge.ReactApplicationContext; import com.facebook.react.bridge.ReactContextBaseJavaModule; import com.facebook.react.bridge.ReactMethod;
public class AndroidModule extends ReactContextBaseJavaModule { AndroidModule(ReactApplicationContext context) { super(context); }
@Override
public String getName() {
return “AndroidModule”;
}
@ReactMethod
public void sampleMethod() {
// Call the third-party SDK method here
}
}
javascript // index.js import { NativeModules } from ‘react-native’; const { AndroidModule } = NativeModules;
AndroidModule.sampleMethod();
Leveraging native capabilities through these techniques ensures more efficient resource utilization, leading to improved app performance.
Profile and Optimize Network Requests in React Native
Network optimization is crucial for ensuring smooth app performance. Inefficient network requests can lead to slow loading times and a poor user experience. Here are ways to optimize network requests in React Native:
Reduce Unnecessary Headers and Leverage WebSocket
HTTP requests often carry unnecessary headers that bloat the request size. Minimizing these headers can significantly improve performance. Additionally, for real-time communication, consider using WebSocket instead of traditional HTTP requests.
Example: Reducing Headers js fetch(‘https://api.example.com/data’, { method: ‘GET’, headers: { ‘Content-Type’: ‘application/json’, // Only include necessary headers } });
Example: Using WebSocket js import { WebSocket } from ‘react-native’;
const ws = new WebSocket(‘wss://example.com/socket’);
ws.onopen = () => { console.log(‘Connected to WebSocket’); };
ws.onmessage = (e) => { console.log(e.data); };
ws.onerror = (e) => { console.log(e.message); };
ws.onclose = (e) => { console.log(e.code, e.reason); };
Network Profiling and Debugging Libraries
Profiling network requests helps identify bottlenecks. Two useful libraries are Flipper and Reactotron.
- Flipper: A platform for debugging mobile apps. It supports network inspection, which allows you to see all HTTP traffic between your app and the server.
- Reactotron: A desktop app for inspecting and debugging React Native apps, capable of tracking network requests.
Example: Using Reactotron js import Reactotron from ‘reactotron-react-native’; import { reactotronRedux } from ‘reactotron-redux’;
Reactotron .configure() .use(reactotronRedux()) .connect();
Implementing Request Batching and Prioritization
Batching requests minimizes the number of individual calls to the server, reducing overhead. Also, assigning priorities to requests ensures critical data loads first.
Example: Request Batching js const fetchData = async () => { const [data1, data2] = await Promise.all([ fetch(‘https://api.example.com/data1’), fetch(‘https://api.example.com/data2’) ]);
const json1 = await data1.json(); const json2 = await data2.json();
return { json1, json2 }; };
These techniques help achieve more efficient network operations in your React Native applications, ensuring faster load times and an overall improved user experience.
Conclusion
Implementing the discussed tips in your React Native app can lead to significant performance improvements and a faster app launch. Each strategy, from memoization to network optimization, plays a crucial role in enhancing app speed and providing a seamless user experience.