How to Create Custom Animation in React Native?
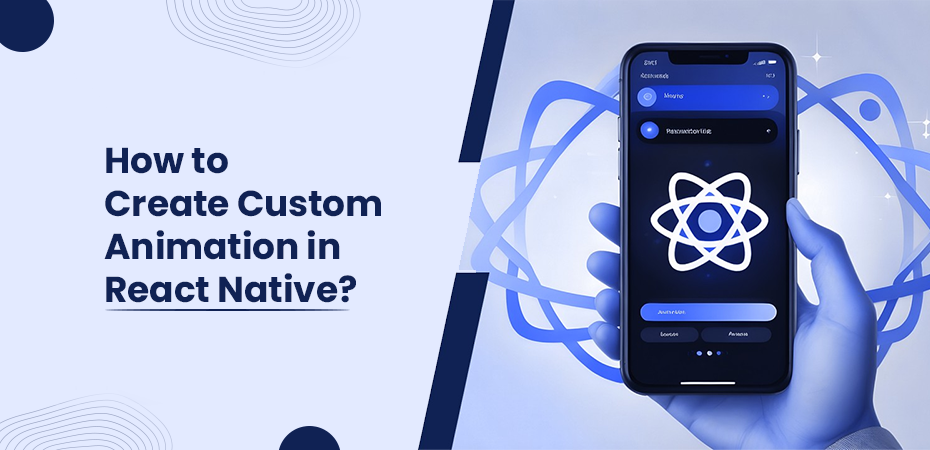
Creating custom animations in React Native is a powerful way to enhance user engagement and improve the overall user experience. By incorporating dynamic animations, developers can make mobile applications more visually appealing and interactive, which is crucial in today’s competitive market. Whether you’re aiming to create smooth transitions, eye-catching text animations, or dynamic live backgrounds anime-style, mastering custom animations can set your app apart.
To achieve these effects, this guide will cover essential tools and libraries that simplify the process:
- Animated API: Provides granular control for complex animations.
- Layout Animation API: Simplifies global layout transitions while running animations.
- React Native Reanimated: Offers advanced features for handling intricate animations.
- React Native Gesture Handler: Enhances touch-based interactions with complex gestures.
Here, in this guide, you will have a solid understanding of how to create custom animations in React Native. This makes your mobile apps not just functional but also visually stunning.
Understanding React Native Animation Systems
The Animated API
Animated API is a powerful system in React Native that provides granular control over animations. It allows developers to create smooth and complex animations by manipulating animated values. You can link these animated values to various properties such as position, scale, and rotation.
Key features of the Animated API include:
- Declarative Animations: Define animations declaratively, making the code cleaner and easier to understand.
- Composability: Combine multiple React animations using Animated.sequence(), Animated.parallel(), or Animated.stagger() for intricate effects.
- Interpolation: Transform animated values using interpolation methods to create non-linear animations.
- Event Handling: Link animations to gestures and events, enabling dynamic reactions to user inputs.
Basic usage involves creating an Animated.Value and then defining an animation type such as Animated.timing(), Animated.spring(), or Animated.decay(). Here is a simple example:
javascript import React, { useRef } from ‘react’; import { Animated, View, Button } from ‘react-native’;
const SimpleAnimation = () => { const fadeAnim = useRef(new Animated.Value(0)).current;
const runAnimation = () => { Animated.timing(fadeAnim, { toValue: 1, duration: 1000, useNativeDriver: true, }).start(); };
return ( <Animated.View style={{ opacity: fadeAnim }}> <View style={{ width: 100, height: 100, backgroundColor: ‘blue’ }} /> </Animated.View> ); };
export default SimpleAnimation;
This example demonstrates how to animate the opacity of a view from 0 to 1 over one second.
Layout Animation
The Layout Animation API provides a way to animate layout changes globally without manually specifying each animation detail. This is particularly useful when you need to animate layout changes resulting from state updates or component re-renders.
Key aspects of Layout Animation include:
- Ease of Use: Simplifies the process of animating layout changes by automatically handling transitions.
- Global Animations: Applies new animations to all layout changes within a specified scope.
- Preset Configurations: Offers predefined configurations for common animation types like ease-in-out.
Using Layout Animation is straightforward. You can configure it with preset options or custom configurations and then run it before updating the state. Here’s an example:
javascript import React, { useState } from ‘react’; import { View, Button, Layout Animation } from ‘react-native’;
const ToggleBox = () => { const [expanded, setExpanded] = use State(false);
const toggleBox = () => { Layout Animation.configureNext(LayoutAnimation.Presets.easeInEaseOut); set Expanded (!expanded); };
return ( <View style={{ height: expanded ? 200 : 100, width: expanded ? 200 : 100, backgroundColor: ‘red’, }} /> ); };
export default ToggleBox;
In this example, pressing the button triggers an animation that smoothly transitions the size of the box between two states. It is done with the help of a preset ease-in-out configuration.
By leveraging both the Animated API and Layout Animation API, developers can create sophisticated and visually appealing animations in their React Native applications.
Essential Libraries for Creating Custom Animations
React Native Reanimated
React Native Reanimated offers advanced capabilities over the default Animated API, especially when dealing with complex animations. This library provides more fluid and performant animations by leveraging the native thread. It ensures that your animations run smoothly without dropping frames.
Key features of React Native Reanimated include:
- Declarative Syntax: It allows you to write animations in a more declarative way, making your code cleaner and easier to understand.
- Native Driver: By utilizing the native driver, animations are offloaded to the native thread, resulting in better performance.
- Enhanced Interactions: Complex gestures and interactions can be handled more efficiently. For instance, creating smooth swiping actions on a FlatList react native or animating a react-native image is easier with Reanimated.
To get started with React Native Reanimated:
bash npm install react-native-reanimated
Additional Libraries
Several other libraries complement React Native Reanimated by enhancing animation capabilities and handling specific use-cases:
React Native Animatable
React Native Animatable simplifies the process of adding predefined animations to your components. This library comes with a variety of built-in animations that can be easily applied to standard components like buttons, images, or text fields.
Benefits of using React Native Animatable:
- Ease of Use: Quickly add animations without writing custom animation code.
- Variety of Animations: Includes a wide range of animations such as fades, bounces, and slides.
Install it via npm:
bash npm install react-native-animatable
Gesture Handler
React Native Gesture Handler enhances touch-based interactions within your app. This library provides a robust gesture system that works seamlessly with both simple and complex gestures. This makes it ideal for creating sophisticated user interactions.
Why use Gesture Handler:
- Better Gesture Handling: Offers improved gesture performance and flexibility over the default gesture responder system.
- Integration with Reanimated: Works well alongside React Native Reanimated for smooth and responsive animations during gestures.
Install it using npm:
bash npm install react-native-gesture-handler
Using these libraries together can significantly improve the animation capabilities of your React Native application. This allows you to create engaging and visually appealing user interfaces.
Setting Up Your Project for Custom Animations
Creating custom animations in React Native dropdown starts with setting up your project correctly and installing the necessary libraries. This section covers the essential steps to get your project ready for implementing animations.
Steps to Create a New React Native Project
1. Install Node.js and Watchman:
- Ensure you have Node.js installed. You can download it from the official website.
- For macOS users, install Watchman using Homebrew: bash brew install watchman.
2. Create a New React Native Project:
- Use the React Native CLI to create a new project. Open your terminal and run: bash npx react-native init MyAnimatedApp cd MyAnimatedApp
Installing Necessary Libraries
To implement custom animations, you’ll need to install specific libraries such as react-native-reanimated and react-native-gesture-handler. These libraries provide robust tools for creating complex animations and handling gestures.
Install React Native Reanimated:
- Run the following command in your project directory: bash npm install react-native-reanimated
- Link the library if you’re not using Expo: bash npx pod-install ios
Install React Native Gesture Handler:
- Run the command: bash npm install react-native-gesture-handler
- Again, for non-Expo projects, link it: bash npx pod-install ios
Configure Babel for Reanimated:
- Add the Reanimated plugin to your Babel configuration (babel.config.js): javascript module.exports = { presets: [‘module:metro-react-native-babel-preset’], plugins: [ ‘react-native-reanimated/plugin’, ], };
Wrap Your Application with Gesture Handler:
- Modify your entry point (usually App.js) to wrap your application with GestureHandlerRootView provided by react-native-gesture-handler: javascript import ‘react-native-gesture-handler’; import { GestureHandlerRootView } from ‘react-native-gesture-handler’;
- const App = () => ( <GestureHandlerRootView style={{ flex: 1 }}> {/* Your app components */} );
- export default App;
Following these steps will set up your React Native project with the necessary dependencies for creating custom animations. This foundation is crucial as it ensures that you have access to advanced animation capabilities and gesture handling features provided by these powerful libraries.
Customizing Animation Parameters for Desired Effects
Customizing animation parameters allows you to achieve specific effects that can significantly enhance the user experience. Key parameters such as easing functions, durations, and delays play a crucial role in defining how animations behave.
Easing Functions
Easing functions determine the acceleration and deceleration of an animation. They can make movements appear more natural by mimicking real-world physics. React Native provides several built-in easing functions, such as:
- Easing.linear: Moves at a constant speed.
- Easing.ease: Starts slow, speeds up, then slows down.
- Easing.bounce: Mimics a bouncing effect.
To apply an easing function, you use it within an animation configuration. For example:
javascript import { Animated, Easing } from ‘react-native’;
Animated.timing(animatedValue, { toValue: 1, duration: 1000, easing: Easing.bounce, useNativeDriver: true, }).start();
Durations
The duration parameter defines how long an animation takes to complete. This is specified in milliseconds. Shorter durations create snappier animations, while longer durations provide a more gradual transition.
Example:
javascript Animated.timing(animatedValue, { toValue: 1, duration: 500, // half a second useNativeDriver: true, }).start();
Delays
Delays introduce a pause before starting an animation. This can be useful for sequencing multiple animations or creating staggered effects.
Example:
javascript Animated.timing(animatedValue, { toValue: 1, duration: 1000, delay: 300, // starts after a delay of 300ms useNativeDriver: true, }).start();
Combining Parameters
Combining these parameters allows for highly customized animations:
javascript Animated.timing(animatedValue, { toValue: 1, duration: 1500, easing: Easing.inOut(Easing.quad), // combination of in and out easing delay: 200, useNativeDriver: true, }).start();
Implementing Animated Components in Your App
To create custom animations in React Native, you need to convert standard components into animated components. This is achieved by using Animated.createAnimatedComponent().
Converting Standard Components
1. Import the Necessary Modules:
javascript import React from ‘react’; import { View, Text } from ‘react-native’; import Animated from ‘react-native-reanimated’;
2. Create Animated Versions of Components:
javascript const AnimatedView = Animated.createAnimatedComponent(View); const AnimatedText = Animated.createAnimatedComponent(Text);
3. Use the Animated Components:
javascript const MyAnimatedComponent = () => { return ( Animating Text! ); };
Applying Animated Styles
To apply animated styles, you need to define them using animation logic and then attach them to your animated components.
1. Define Animation Logic:
javascript const translation = useSharedValue(0);
const animatedStyles = useAnimatedStyle(() => { return { transform: [{ translateY: translation.value }], }; });
2. Attach Styles to Components:
javascript const MyAnimatedComponent = () => { return ( Animating Text! ); };
This process allows you to animate properties like position, scale, rotation, and opacity. For instance, animating a component’s position can be achieved by adjusting its translateY value over time.
Example: Animating a Button
Consider an example where we animate a button click:
1. Define the Button Component:
javascript const AnimatedButton = () => { const scale = useSharedValue(1);
const animatedStyles = useAnimatedStyle(() => { return { transform: [{ scale: scale.value }], }; });
const handlePressIn = () => { scale.value = with react-Spring(0.8); };
const handlePressOut = () => { scale.value = withSpring(1); };
return ( <Animated.View style={[styles.button, animatedStyles]}> Press Me </Animated.View> ); };
const styles = StyleSheet.create({ button: { backgroundColor: ‘#6200ea’, padding: 10, borderRadius: 5, alignItems: ‘center’, }, });
By following these steps, you can effectively implement animated components within your React Native app, creating dynamic and engaging user experiences.
This approach ensures that your animations are smooth and responsive, leveraging React Native’s powerful animation libraries like Reanimated and Gesture Handler.
Using Hooks for Managing Component Behaviors During Animations
React Native Reanimated introduces powerful hooks that simplify managing component behaviors during animations. One such hook is useAnimatedStyle, which plays a crucial role in applying images in animated styles directly to components.
Understanding useAnimatedStyle
useAnimatedStyle allows you to define and apply styles that change dynamically based on animation values. This hook returns an animated style object that can be used with the Animated.View or any other animated component.
Benefits of useAnimatedStyle
- Performance: By leveraging the native driver, useAnimatedStyle ensures smooth and high-performance animations by offloading computations to the native thread.
- Simplicity: It simplifies the process of creating complex animations by allowing direct manipulation of styles within a functional component. You can create an animated background on your phone by applying this technique.
- Reactivity: Styles defined with useAnimatedStyle are reactive, meaning they automatically update when the animation values change.
Performance Considerations for Smooth User Experiences
Benefits of Utilizing the Native Driver
To achieve smooth interactions and optimal performance in React Native animations, leveraging the native driver is crucial. The native driver offloads animation calculations to the native thread instead of the JavaScript thread. It ultimately results in more fluid animations.
- Reduced Lag: By using the native driver, animations run independently from JavaScript execution, reducing lag. They ensure that UI transitions remain smooth even under heavy computational loads.
- Better Frame Rates: Offloading work to the native thread helps maintain higher frame rates, which is essential for a seamless user experience.
Optimizing Frame Rates
Maintaining high frame rates ensures that animations appear smooth and natural. Here are some tips for optimizing frame rates:
- Minimize Heavy Computations: Reduce complex computations on the JavaScript thread during animations. Offload these tasks where possible.
- Use useNativeDriver: Enable useNativeDriver in your animations configuration. For example: javascript Animated.timing(animatedValue, { toValue: 1, duration: 500, useNativeDriver: true }).start();
- Monitor Performance: Use tools like React Native Performance Monitor to identify bottlenecks, and text animations in CSS, and optimize accordingly.
Handling Media Components
Animations involving media elements like images, videos, or sliders can be demanding on performance. Here’s how to manage them effectively:
React Native Picture and Video
When animating media components like pictures or videos (react-native-video), ensure they are preloaded and optimized for performance.
Example for animated video component: javascript import Video from ‘react-native-video’;
<Animated.View style={animatedStyle}> <Video source={{uri: ‘path-to-video’}} /> </Animated.View>
React Native Slider
For components like sliders where continuous state changes occur, debounce updates to avoid excessive re-renders.
Example: javascript import Slider from ‘@react-native-community/slider’;
<Slider style={{width: 200}} minimumValue={0} maximumValue={100} onValueChange={debouncedHandleChange} />
Conclusion
Exploring custom animation possibilities in React Native projects shows numerous ways to enhance user experience. By leveraging the best tools for React Native animations, such as Reanimated and Gesture Handler, developers can create visually appealing and responsive interfaces.
Custom animations significantly impact user engagement by adding interactivity and smooth transitions to applications. As these tools and libraries continue to evolve, expect future trends to push the boundaries of what’s possible in mobile app animation.
Embrace these techniques to not only improve your current projects but also stay ahead in delivering cutting-edge user experiences.