Integrating Third-Party Libraries in React Native Framework
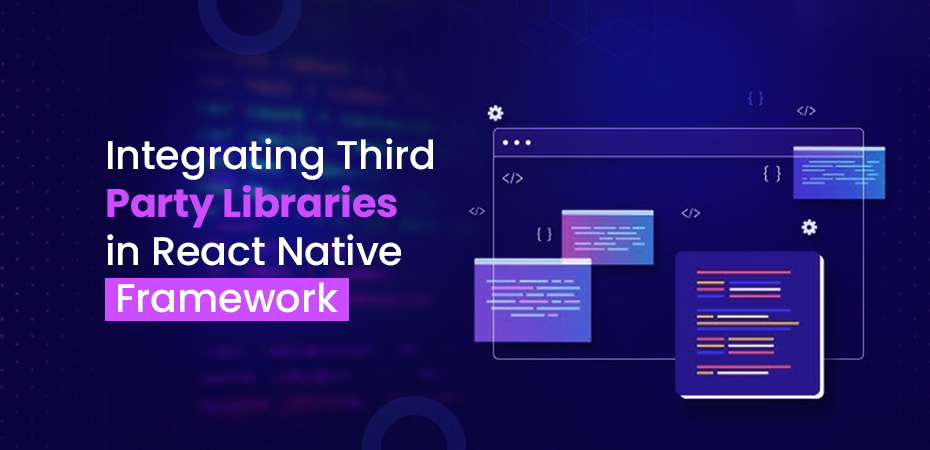
Integrating third-party libraries in React Native is a crucial practice that significantly improves the functionality of applications. By using these libraries, developers can expand the built-in capabilities of React Native to include advanced features and functionalities without having to create them from scratch. This method not only speeds up development time but also ensures the app remains strong and scalable.
Here, in this blog, you will learn:
- Provide a comprehensive understanding of integrating third-party libraries in React Native.
- Offer step-by-step instructions on installing and managing these libraries.
- Highlight popular libraries for various use cases, ensuring developers make informed choices.
- Discuss best practices and considerations to ensure seamless integration and optimal performance.
Whether you’re looking to incorporate UI components, handle navigation, manage forms, or enhance networking capabilities, this guide will equip you with the necessary knowledge to effectively integrate third-party libraries in your React Native projects. Expect to learn about tools like npm install react js, react native link, yarn add dev dependency, and more, as well as insights into popular libraries such as npmjs lodash.
Understanding Third-Party Libraries in React Native
Third-party libraries are pre-packaged code modules developed by the community or individual developers that extend the built-in capabilities of React Native. These libraries can significantly speed up development by providing ready-to-use components and functionalities, allowing developers to focus more on building unique features rather than reinventing the wheel.
Role of Third-Party Libraries
- Enhancement: They enhance the application’s functionality by adding features not natively available in React Native.
- Efficiency: Utilization of these React libraries can reduce development time and improve code quality.
- Standardization: Common tasks such as form validation, data fetching, and navigation are standardized through these libraries.
Popular Sources for Finding Libraries
NPM (Node Package Manager):
As the largest repository of JavaScript packages, NPM is a go-to source for finding third-party libraries. React Native developers can search for specific functionalities or explore trending packages. You will know about total npm downloads. Example: To install lodash npm, a popular utility library, you would run: bash npm install lodash. You can check its popularity via total npm lodash downloads.
React Native Directory:
A curated database specifically for React javascript libraries, making it easier to find libraries that work seamlessly with your project. Example: Searching for react-native-webview will provide details on how to integrate web views into your app.
GitHub:
Many third-party libraries are hosted on GitHub where you can access their repositories, documentation, and community support. Example: For integrating vector icons using react-native-vector-icons, you can find the repository and installation instructions on GitHub.
Examples of Popular Libraries
- UI Libraries: Enhance user interfaces with additional components like NativeBase and Material UI.
- Navigation Libraries: Manage navigation easily with packages like React Navigation.
- Networking Libraries: Simplify API calls using tools like Axios or Apollo Client.
Utilizing these resources ensures that your React Native applications are robust, feature-rich, and maintainable.
Ensuring Compatibility of Third-Party Libraries
Checking library compatibility with different React Native versions and platforms is crucial before integration. An incompatible library can lead to unexpected bugs, crashes, or degraded performance in your application. You might be thinking what are third-party libraries used in React JS framework?
Importance of Library Compatibility
- React Native Versioning: React Native component library frequently updates, introducing new features, deprecations, and bug fixes. The best React select component Libraries must keep pace with these changes to maintain compatibility.
- Platform-Specific Considerations: Some libraries may work seamlessly on iOS but face issues on Android, or vice versa. Ensuring compatibility across both platforms is essential for a consistent user experience.
Methods for Testing Compatibility
Review Documentation and Release Notes:
- Check the library’s documentation for supported React Native versions.
- Look at release notes for any mention of compatibility updates or issues.
Community Feedback:
- Visit forums like Stack Overflow or GitHub Issues to see if other developers have reported compatibility problems.
- Engage with the community to ask direct questions about experiences with specific library versions.
Trial and Error:
- Install the library in a controlled environment (e.g., a separate branch) to test its behavior.
- Run your application on both iOS and Android simulators/emulators to identify any platform-specific issues.
Automated Testing:
- Implement unit and integration tests that cover critical parts of your app using the third-party library.
- Use Continuous Integration (CI) tools to run these tests across different environments automatically.
Version Management Tools:
- Utilize tools like npm and Yarn workspaces to manage dependencies and ensure you are using compatible versions. Learn Yarn how to uninstall plugin.
- Leverage package.json to specify compatible library versions explicitly.
json { “dependencies”: { “react-native”: “^0.64.0”, “some-library”: “^1.2.3” } }
Ensuring library compatibility before integrating third-party libraries in React Native not only prevents potential issues but also saves time in troubleshooting later stages of development. This proactive approach lays a solid foundation for building robust and dependable applications.
Popular Third-Party Libraries for Various Use Cases in React Native Development
1. UI Libraries
UI libraries are essential for building visually appealing and user-friendly applications. By integrating third-party UI libraries, developers can leverage pre-built components and design systems to accelerate development and maintain consistency across the app.
Popular UI Libraries
1. Lottie
Lottie is a popular library for adding animations to React Native applications. Developed by Airbnb, it uses JSON files exported from Adobe After Effects to render high-quality animations in real-time.
- Installation: bash npm install –save lottie-react-native
- Usage Example: jsx import React from ‘react’; import LottieView from ‘lottie-react-native’;
const AnimationComponent = () => ( <LottieView source={require(‘./animation.json’)} autoPlay loop /> );
export default AnimationComponent;
2. Material UI
Material UI provides a comprehensive set of UI components following Google’s Material Design guidelines. While it is primarily used in web development with React, there are equivalents like react-native-paper that offer similar functionality for React Native.
- Installation: bash npm install react-native-paper
- Usage Example: jsx import * as React from ‘react’; import { Button, Card } from ‘react-native-paper’;
const MyComponent = () => ( <Card.Title title=”Card Title” subtitle=”Card Subtitle” /> <Card.Content> Press me </Card.Content> );
export default MyComponent;
2. Navigation Libraries
Navigation is a core component of mobile applications, enabling users to move seamlessly between different screens and features. In React Native, navigation libraries play a crucial role in creating smooth and intuitive user experiences.
Popular Navigation Libraries:
- React Navigation: Widely regarded as the go-to library for navigation in React Native apps, React Navigation offers a variety of navigators such as stack, tab, and drawer navigators. Its flexible API and extensive documentation make it an excellent choice for handling complex navigation patterns.
3. Form Management Libraries
Handling user input efficiently is crucial in any application, and Form Management Libraries in React Native simplify this process significantly. These libraries facilitate the management of form state, validation, and submission, reducing boilerplate code and making forms more maintainable.
Popular Form Management Libraries
Formik and Redux Form are two highly popular libraries in this domain:
- Formik: Known for its simplicity and ease of use, Formik helps manage form state, handle validation, and submit forms with minimal setup. It integrates seamlessly with React Native components, allowing developers to create complex forms quickly.
- Redux Form: This library leverages Redux to manage form state globally. It provides powerful tools for form validation and submission while ensuring the form state is synchronized with the application’s state.
4. Specialized Libraries
Third-party libraries play a crucial role in handling specific functionalities within React Native applications. These specialized libraries extend the core capabilities of the framework, enabling developers to implement complex features with ease.
Here are some categories and examples of specialized libraries:
- In-App Purchases: Handling transactions within mobile applications is simplified with libraries such as react-native-iap. This library provides APIs for both iOS and Android platforms, making it easier to integrate in-app purchases seamlessly.
- AR/VR Development: Augmented reality and virtual reality experiences can be crafted using ViroReact. This library supports creating immersive AR/VR applications that can run across different devices, bringing innovative features to your app.
- Media Handling: For managing media content like images and videos, react-native-video and react-native-image-picker are popular choices. These libraries offer robust solutions for media playback and user media selection.
- Animations: Libraries like Lottie enable developers to add high-quality animations to their apps effortlessly. By using JSON-based animations created in Adobe After Effects, Lottie enhances the visual appeal of your applications.
- Localization: To cater to a global audience, localization libraries such as react-native-localize help manage multi-language support within applications. This ensures that users receive content in their preferred language, enhancing user experience.
Leveraging these specialized third-party libraries allows React Native developers to incorporate advanced functionalities without reinventing the wheel. By utilizing well-maintained and documented libraries, development becomes more efficient and focused on delivering value to end-users.
Documentation Quality and Community Support in Choosing Third-Party Libraries
When integrating third-party libraries into a React Native project, the quality of library documentation is paramount. Thorough documentation serves as a roadmap for developers, guiding them through installation, configuration, and usage. Comprehensive documentation typically includes:
- Installation Instructions: Detailed steps for setting up the library using npm or Yarn add dev deps.
- Configuration Guidelines: Tips on configuring the library for different platforms (iOS/Android). Learning about installing React with npm framework.
- Usage Examples: Code snippets demonstrating typical use cases.
- API Reference: Detailed descriptions of all available functions and properties.
Library documentation importance cannot be overstated. It can significantly reduce the learning curve, enabling quicker integration and troubleshooting. For instance, well-documented libraries like React Navigation offer step-by-step guides that simplify complex setups.
Conclusion
Integrating third-party libraries in React Native can transform your development process by leveraging existing solutions. These libraries enhance functionality, streamline workflows, and open up new possibilities. Engage with other developers on platforms like Stack Overflow or Reddit.
Check out curated lists such as the React Native Directory to discover tools that align with your project needs. Harness the power of third-party libraries to create robust, feature-rich applications effectively while maintaining a focus on performance and user experience.