React Native End-to-End Testing Using Detox
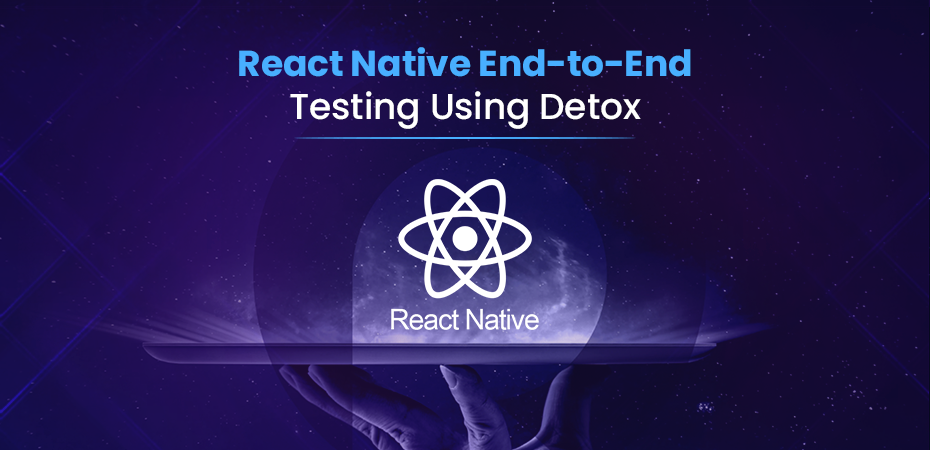
End-to-end (E2E) testing is a critical part of the software development lifecycle, ensuring that all components of an application work together seamlessly from the user’s perspective. Unlike unit testing, which focuses on individual components, or integration testing, which tests interactions between components, E2E testing simulates real-world user interactions with the entire application. This type of testing is especially important for mobile applications, where user experience is paramount.
However, mobile developers often skip E2E testing due to perceived difficulties in setup and configuration. In this blog, we’ll explore how Detox, an app testing tool, simplifies the testing process for React Native applications and ensures that your app performs reliably across all user scenarios.
Popular Tools for React Native End-to-End Testing
Cypress for Native Testing
Cypress (React Native) is a popular E2E testing tool known for its ease of use and powerful features for web applications. It provides a comprehensive environment for running tests in a browser, offering capabilities like real-time reloading and detailed debugging. However, when it comes to mobile applications, Cypress has limitations. It is primarily designed for web applications, making it less suitable for testing mobile apps that require interaction with native components.
While Cypress excels in testing web and hybrid apps, React Native developers often find themselves needing a more specialized tool for mobile-specific testing.
Detox Application: The Preferred Tool for React Native
Detox, developed by Wix, is an E2E testing framework tailored for mobile applications, particularly React Native. It offers a seamless integration with React Native, allowing developers to write tests in JavaScript using familiar tools like Jest. Detox’s standout feature is its “gray box testing” approach, which reduces test flakiness by ensuring that tests are executed only when the app is idle—meaning all animations, network requests, and timers are completed.
For example, Detox can effectively handle scenarios like testing complex UI components such as the React Native Action Sheet or running tests on asynchronous operations. This makes it a preferred choice for developers looking to implement advanced use cases in their testing process.
React Native Detox (advance use) involves leveraging custom test scripts and integrating with CI/CD pipelines for robust, automated end-to-end testing.
Detox’s integration with React Native makes it the go-to choice for developers looking to ensure their mobile apps perform as expected across various scenarios.
Other Tools and Frameworks
Appium
Appium is another popular tool for mobile automation testing, offering support for a wide range of platforms and languages. It is particularly useful for teams that need to test across different operating systems, as it supports both iOS and Android. However, Appium’s versatility comes with a steeper learning curve, and its tests tend to run slower compared to Detox due to its “black box testing” approach.
Fastlane
Fastlane is not a testing framework but a tool that automates the deployment of mobile apps. However, it integrates well with testing frameworks like Detox, allowing you to automate the entire CI/CD pipeline, including running E2E tests before deploying the app. This integration ensures that your app is rigorously tested and ready for release.
React Native Testing Library
While not a full-fledged E2E testing tool, the React Native Testing Library can be used to test the interaction of components within the app. However, it lacks support for native code, making it less suitable for comprehensive E2E testing. It’s best used in conjunction with tools like Detox to cover all aspects of testing.
Setting Up Detox for React Native
Prerequisites
Before diving into Detox, ensure your development environment is properly set up. You’ll need:
- Xcode (for iOS development)
- Java and Android SDK (for Android development)
- Node.js and Homebrew (for managing packages and dependencies)
Project Initialization
To start, create a new React Native project using the following command:
npx react-native init MyApp
Once your project is set up, install Detox and its dependencies:
npm install detox –save-dev
npm install detox-cli –global
brew tap wix/brew && brew install applesimutils
Initialize Detox in your project with:
detox init -r jest
This command sets up the basic configuration needed for Detox, including configuration files and example tests.
Integrating Detox with CI/CD
To fully leverage Detox, integrate it into your CI/CD pipeline. This ensures that E2E tests are run automatically whenever new code is pushed to the repository, preventing bugs from making it to production. Tools like Travis CI or GitHub Actions can be used to automate this process.
A typical .travis.yml configuration might look like this:
language: objective-c
osx_image: xcode12.5
script:
– npm install
– detox build –configuration ios.sim.debug
– detox test –configuration ios.sim.debug
This setup ensures that your tests are built and executed in a simulated iOS environment every time you push a new commit.
Writing and Running Detox Tests
Basic Detox Test Structure
Detox tests are written in JavaScript, and their syntax is similar to unit tests. Here’s a simple example:
describe('Login Flow', () => { beforeEach(async () => { await device.reloadReactNative(); }); it('should login successfully', async () => { await element(by.id('email')).typeText('test@example.com'); await element(by.id('password')).typeText('password'); await element(by.text('Login')).tap(); await expect(element(by.text('Welcome'))).toBeVisible(); }); });
This test checks that a user can successfully log in by typing in an email and password, tapping the login button, and then verifying that a welcome message appears.
Advanced Test Scenarios
Detox can handle more complex scenarios, such as testing components that involve swiping, dragging, or other gestures. Here’s an example of testing a carousel component:
it('should swipe through the carousel', async () => { await element(by.id('carousel')).swipe('left'); await expect(element(by.text('Second Slide'))).toBeVisible(); await element(by.id('carousel')).swipe('right'); await expect(element(by.text('First Slide'))).toBeVisible(); });
Improving Test Reliability
To reduce flakiness, ensure that your tests are deterministic and handle asynchronous operations properly. Detox’s gray box approach helps by waiting for the app to be idle before performing actions. Additionally, you can configure custom timeouts for operations that might take longer.
Optimizing Detox for Large-Scale React Native Applications
Modularizing Tests
As your application grows, it’s crucial to keep your tests organized. Modularize your tests by breaking them down into smaller, more manageable pieces that focus on specific features or components.
Prioritizing and Parallelizing Tests
Not all tests need to be run every time you push a commit. Prioritize tests based on their importance and frequency of use, and consider running less critical tests less frequently. Detox also supports parallel test execution, allowing you to run multiple tests simultaneously to speed up the process.
Maintaining Test Performance
Regularly update Detox and related dependencies to benefit from performance improvements and bug fixes. Use Fastlane to automate the build and test process, ensuring that your tests are always up to date.
Common Issues and Troubleshooting in Detox
Configuration Errors
One of the common issues with Detox involves configuration errors, especially when setting up the environment. Ensure that all dependencies, like the Android SDK and Xcode, are properly installed to avoid getting stuck on issues like “native access stuck on installing dependencies.”
Flaky Tests and Timeouts
Flaky tests, which pass or fail intermittently, are often caused by race conditions or improper handling of asynchronous code. Make sure your tests are deterministic and handle all async operations correctly. If you encounter timeout errors, consider increasing the allowed time or optimizing the app’s performance.
Detox Alternatives and Complementary Tools
Comparison of Detox with Appium, Cypress, and Jest
While Detox is specifically designed for React Native, it’s not the only tool available. Appium is versatile but slower, and Cypress, in native mobile testing, is not a good choice though it excels in web testing. Jest is ideal for unit tests, but for E2E, it often requires integration with other tools like Detox.
Using Fastlane for E2E Testing Automation
Fastlane complements Detox by automating the build and deployment process. This is particularly useful in large projects where continuous delivery is key to maintaining product quality.
Conclusion
Detox is a powerful tool for E2E testing in React Native applications, offering robust features that integrate seamlessly with your development workflow. Its ability to handle complex test scenarios and reduce flakiness makes it an essential tool for ensuring the reliability of your mobile apps.
Integrating Detox with Fastlane and other tools into your CI/CD pipeline can further enhance your testing process, ensuring that your app is always ready for production. By following the steps and best practices outlined in this blog, you can set up a comprehensive E2E testing strategy of app by detox that ensures your React Native end app delivers a flawless user experience.