React Native vs Bootstrap- Which is better in 2024
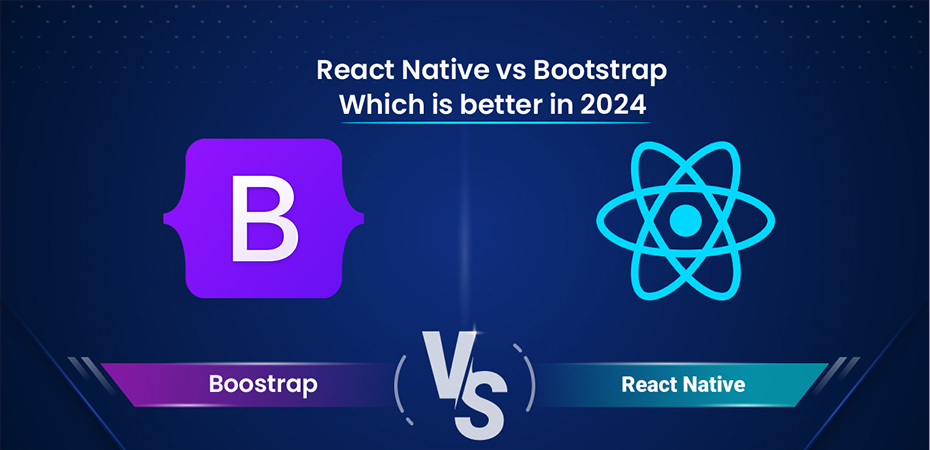
React and Bootstrap are two of the most popular front-end frameworks in web development today. React is a JavaScript library developed by Facebook, designed for building interactive user interfaces with a component-based architecture. It excels in creating highly efficient web applications through its virtual DOM and reusability of components.
Bootstrap, on the other hand, is an open-source framework initially created by Twitter. It offers HTML, CSS, and JavaScript-based design components that facilitate the creation of responsive and visually appealing applications. Its flexibility and rapid prototyping capabilities have made it a go-to choice for developers aiming to build consistent UI designs quickly.
Understanding both React and Bootstrap is crucial in modern web development due to their distinct yet complementary roles:
- React: Enhances functionality through dynamic UIs and scalability.
- Bootstrap: Ensures quick, responsive design and maintains consistency across projects.
Understanding React
React, a popular JavaScript library developed by Facebook, revolutionizes the way developers build user interfaces. It emphasizes a component-based architecture and leverages the virtual DOM to create highly efficient web applications.
Key Features of React
1. Virtual DOM
React uses a virtual representation of the DOM to optimize updates and rendering. Instead of updating the real DOM directly, React makes changes to the virtual DOM first. It then calculates the most efficient way to update the real DOM, which significantly improves performance.
2. Component Reusability
Components are the building blocks in React. They encapsulate specific parts of a UI, making them reusable across different parts of an application. This modularity allows for cleaner code and easier maintenance.
Advantages of Using React in Web Applications
1. Performance Optimization Techniques
- Code Splitting: Breaks down large bundles into smaller chunks, improving load times.
- Lazy Loading: Loads components only when they’re needed, enhancing initial load performance.
- Memoization: Caches results of expensive function calls to avoid redundant calculations.
2. Cross-Platform Compatibility
- React Native: Allows you to build mobile applications using React principles, leveraging a single codebase for both iOS and Android platforms.
- Server-Side Rendering (SSR): Improves SEO and initial load performance by rendering components on the server side.
3. Strong Community Support
- Extensive libraries and tools: The React ecosystem includes numerous libraries like Redux for state management and Next.js for server-side rendering.
- Vibrant community: Developers can easily find solutions, tutorials, and best practices through forums like Stack Overflow, GitHub repositories, and official documentation.
React’s component-based approach and virtual DOM bring significant benefits in terms of performance and reusability. Its strong community support ensures continuous improvement and access to a wealth of resources.
Exploring Bootstrap
History and Evolution of Bootstrap
Bootstrap, originally called Twitter Blueprint, was created by Mark Otto and Jacob Thornton at Twitter as a framework to ensure consistency across internal tools. It was first released to the public in August 2011 and quickly became popular because of its wide range of design components and user-friendly interface.
Since then, Bootstrap has undergone significant updates:
- Bootstrap 3: Introduced a mobile-first approach, changing the way responsive design is done.
- Bootstrap 4: Added more flexibility with a grid system based on Flexbox, improved utilities, and better customization options.
- Bootstrap 5: Removed the need for jQuery, improved support for CSS grid, and focused on modern HTML and CSS standards.
Advantages of Bootstrap for Developers
Bootstrap offers several advantages that make it a preferred choice for web developers:
- Flexibility: With a wide range of pre-designed components like navigation bars, forms, buttons, and modals, Bootstrap provides a flexible foundation for building UI UX designs. Developers can customize these components to fit specific project needs without starting from scratch.
- Rapid Prototyping: The ready-to-use code snippets and extensive documentation enable developers to quickly prototype ideas. This rapid prototyping capability is crucial for iterating designs efficiently during the development process.
- Responsive Design: Bootstrap’s grid system ensures that websites are inherently responsive. By using a series of containers, rows, and columns, developers can create layouts that adapt seamlessly across different screen sizes.
- Accessibility Compliance: Accessibility is built into Bootstrap’s core design philosophy. Components are designed with ARIA (Accessible Rich Internet Applications) attributes and roles to ensure web applications are accessible to users with disabilities.
- Consistency: Using Bootstrap ensures a consistent look and feel across different web pages and projects. This consistency is essential for maintaining brand identity and providing a cohesive user experience.
- Community Support: As an open-source project with strong community backing, Bootstrap benefits from regular updates and improvements. Developers can access extensive resources including documentation, tutorials, forums, and third-party plugins.
The combination of flexibility, rapid prototyping capabilities, and built-in accessibility compliance makes Bootstrap an essential tool in modern web development.
Comparing React and Bootstrap
When to Use React for Dynamic Functionality vs. When to Use Bootstrap for Rapid Prototyping
React and Bootstrap serve distinct yet complementary roles in modern web development. Understanding when to leverage each can significantly enhance your project outcomes.
Advantages and Disadvantages of React and Bootstrap:
React:
Advantages:
- Component-based architecture
- Efficient rendering through Virtual DOM
- High reusability of code
- Extensive ecosystem and community support
Disadvantages:
- Steep learning curve for beginners
- Complex setup for larger-scale projects
Bootstrap:
Advantages:
- Flexibility and ease of use
- Time-saving with pre-styled components
- Accessibility compliance
Disadvantages:
- Heavy loading time and battery drain on mobile devices with poor internet connections
- Limited style customization, leading to similar-looking sites
Differences between React and Bootstrap:
Purpose:
- React focuses on building dynamic user interfaces with reusable components.
- Bootstrap excels in rapid prototyping with a consistent, responsive design framework.
Functionality:
- Use React when your project requires interactive, state-driven UIs, such as single-page applications (SPAs) or complex user interfaces.
- Opt for Bootstrap when you need to quickly create a responsive website layout, especially useful during initial project phases.
Combining Strengths:
In many cases, combining the strengths of both frameworks can yield optimal results. Utilize React’s powerful component system to manage dynamic content while leveraging Bootstrap’s CSS framework for rapid UI development. This hybrid approach allows you to maintain high performance without compromising on design aesthetics.
Understanding these nuances ensures you select the right tool based on specific project requirements, enhancing both efficiency and effectiveness in web development.
Using React and Bootstrap Together
How to Integrate React with Bootstrap
Integrating React components with Bootstrap CSS can enhance your project’s efficiency and aesthetics. Here’s how you can do it:
Install Bootstrap: First, you need to install Bootstrap in your React project. You can do this using npm:
bash npm install bootstrap
Import Bootstrap CSS: Next, import the Bootstrap CSS into your index.js or App.js file:
javascript import ‘bootstrap/dist/css/bootstrap.min.css’;
Use Bootstrap Classes: With this setup, you can start using Bootstrap classes within your React components. For example, creating a simple button component with Bootstrap styling:
javascript import React from ‘react’;
const MyButton = () => ( Click Me! );
export default MyButton;
This approach leverages the power of both frameworks by combining Bootstrap’s robust styling capabilities with React’s dynamic functionality.
Best Practices for Combining the Strengths of Both Frameworks
To get the most out of integrating React with Bootstrap, consider these best practices:
1. Component-Based Implementation
Break down your UI into reusable components. Use Bootstrap classes to style these components efficiently.
2. Avoid Inline Styles
Use Bootstrap’s predefined classes instead of inline styles to maintain consistency and leverage responsive design features.
3. Use React-Bootstrap Library
For seamless integration, consider using the react-bootstrap library. It provides Bootstrap components as React components, ensuring better compatibility and easier implementation:
bash npm install react-bootstrap bootstrap
Example usage:
javascript import React from ‘react’; import { Button } from ‘react-bootstrap’;
const MyButton = () => ( Click Me! );
export default MyButton;
4. Code Splitting
Implement code-splitting techniques to load only necessary components and styles. This reduces initial load times and enhances performance.
5. Custom Themes
Customize Bootstrap themes to align with your project’s branding. This approach ensures that your application maintains a unique look while benefiting from Bootstrap’s responsive capabilities.
By following these guidelines, you can effectively combine the strengths of both frameworks, resulting in a well-structured and highly functional web application.
Performance Considerations When Using Both Frameworks Together
Optimizing performance when using React and Bootstrap together requires a thoughtful approach. Here’s how you can enhance load times and mitigate rendering issues:
Techniques for Performance Optimization
- Code Splitting: Use tools like Webpack to split your code into smaller chunks. This helps in loading only the necessary components, reducing initial load times.
- Lazy Loading: Implement lazy loading for React components and Bootstrap resources. This ensures that only essential parts of the application are loaded initially, with less critical elements being loaded as needed.
- Minification: Minify your CSS and JavaScript files to decrease file sizes. Tools like UglifyJS and CSSNano can help compress your code, leading to faster load times.
- Tree Shaking: Remove unused code from your final bundle using tree shaking techniques available in module bundlers like Webpack.
- Server-Side Rendering (SSR): Leverage server-side rendering to improve the initial load performance of your application. SSR can serve fully rendered pages from the server, making the user experience smoother.
- CSS-in-JS: Consider using CSS-in-JS libraries like styled-components or Emotion instead of traditional CSS frameworks to reduce the amount of CSS that needs to be loaded.
- Caching Strategies: Implement effective caching strategies for both static assets and dynamic content. Use service workers and HTTP caching headers to store frequently accessed resources locally.
By combining these techniques, you can strike a balance between leveraging the strengths of both React and Bootstrap while maintaining optimal performance in your web applications.
Future Trends in Web Development with React and Bootstrap
Predictions on the Evolving Relationship Between These Two Technologies
The future of front-end frameworks like React and Bootstrap indicates an increasingly symbiotic relationship. As web development trends shift towards even more dynamic and responsive user interfaces, both technologies are expected to evolve to better serve these demands.
1. Enhanced Integration Capabilities
- Developers may see tighter integration tools between React components and Bootstrap styles, simplifying the process of combining the two.
- Expect more libraries and plugins designed specifically to bridge gaps between these frameworks.
2. Focus on Performance
- As performance optimization remains crucial, advancements in both React and Bootstrap will likely address load times and rendering issues when used together.
- Techniques like code splitting, tree shaking, and server-side rendering might be more seamlessly incorporated.
3. Component Customization
- Customizability will be a key trend, with Bootstrap potentially offering more flexible design components that can be easily tailored to fit within a React structure.
- Look out for component libraries that leverage the strengths of both frameworks for rapid yet unique UI development.
4. AI-Driven Enhancements
AI is transforming many aspects of web development; integration of AI capabilities within React and Bootstrap could provide new tools for predictive design adjustments and automated performance tuning.
5. Growing Ecosystem Support
- The community around these frameworks will continue to expand, contributing new tools, resources, and best practices that enhance their combined usage.
- More educational content and documentation aimed at helping developers navigate using both technologies together efficiently.
- The evolving landscape of web development promises exciting advancements, making it crucial to stay updated with the latest trends involving React and Bootstrap.
Conclusion & Next Steps
Choosing the right tools based on your project requirements is essential. React and Bootstrap play important roles in web development, enhancing both functionality and design.
- React offers dynamic UI capabilities and scalability.
- Bootstrap provides rapid prototyping and ensures consistency.
Experimenting with both frameworks can lead to significant improvements in your web development skills. By using their strengths, you can create responsive, efficient, and visually appealing applications.